もし住所から経度と緯度を取得する必要がある場合、以下のようなコードを使ってGoogle Maps Geocoding APIを使用することができます。
pythonです↓
import requests
def get_lat_lng(address, api_key):
url = f"https://maps.googleapis.com/maps/api/geocode/json?address={address}&key={api_key}"
response = requests.get(url)
result = response.json()
if result['status'] == 'OK':
location = result['results'][0]['geometry']['location']
return location['lat'], location['lng']
else:
return None, None
# 使用例
api_key = 'YOUR_API_KEY'
address = '新宿区西新宿2-8-1'
lat, lng = get_lat_lng(address, api_key)
print(f'緯度: {lat}, 経度: {lng}')
というお達しがありましたが。
以下のコードでやっとエクセルファイルが読み込めたのでまたの機会に。
大分時間かかったんですが、そもそものExcelに緯度と経度を入れていなかったということで自分に非しかない落ち度です。
カラムが足りませんというアナウンスじゃ全然ぴんと来なかった。。PINだけに。。
以下がエクセルファイルからの抽出
ピン表示はOK
ピンをクリックするとタイトルが出ます。
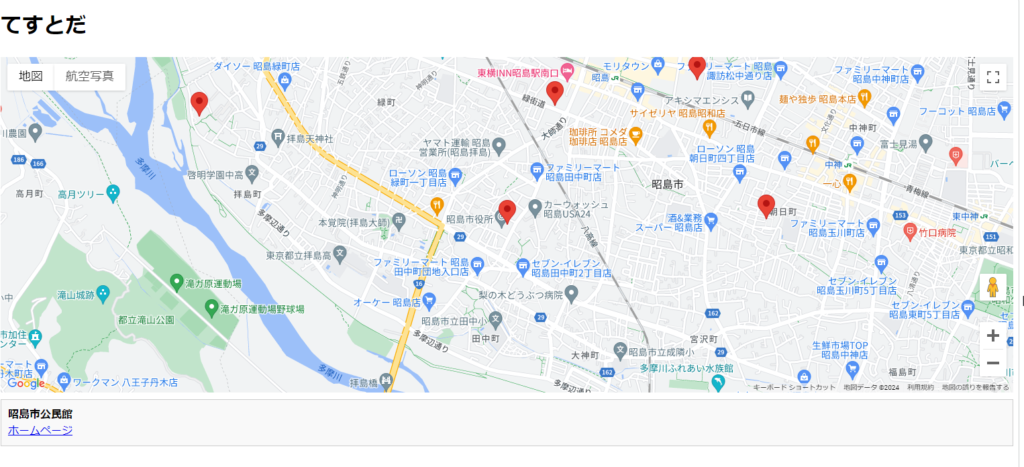
import pandas as pd
# Excelファイルのパスを指定
file_path = r"C:\mapion_sc\mapionNext.xlsx"
# Excelファイルを読み込む
try:
df = pd.read_excel(file_path)
print(df.info())
print(df.head())
except Exception as e:
print(f"エラー: {e}")
df = pd.DataFrame() # 空のDataFrameを作成しておく
# HTMLファイルの作成
html_content = '''
<!DOCTYPE html>
<html>
<head>
<title>てすてす</title>
<script src="https://maps.googleapis.com/maps/api/js?key=YourAPIkey"></script>
<style>
#map {
height: 500px;
width: 100%;
}
#info {
margin-top: 10px;
padding: 10px;
border: 1px solid #ccc;
background-color: #f9f9f9;
}
</style>
</head>
<body>
<h1>てすとだ</h1>
<div id="map"></div>
<div id="info">ここに情報が表示されます。</div>
<script>
function initMap() {
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 10,
center: {lat: 35.681236, lng: 139.767125} // 初期位置を東京に設定
});
var locations = [
'''
# データをHTMLに追加
for index, row in df.iterrows():
try:
url = row.iloc[0] if pd.notna(row.iloc[0]) else ''
title = row.iloc[1] if pd.notna(row.iloc[1]) else ''
lat = row.iloc[2] if pd.notna(row.iloc[2]) else 0
lng = row.iloc[3] if pd.notna(row.iloc[3]) else 0
html_content += f'''
{{
url: "{url}",
title: "{title}",
lat: {lat},
lng: {lng}
}}{',' if index < len(df) - 1 else ''}
'''
except Exception as e:
print(f"行 {index} でエラーが発生しました: {e}")
# 最後の閉じかっこを追加
html_content += '''
];
locations.forEach(function(location) {
var marker = new google.maps.Marker({
position: {lat: location.lat, lng: location.lng},
map: map,
title: location.title
});
marker.addListener('click', function() {
map.setZoom(15);
map.setCenter(marker.getPosition());
document.getElementById('info').innerHTML =
`<strong>${location.title}</strong><br>
<a href="${location.url}" target="_blank">ホームページ</a>`;
});
});
}
</script>
<script>
window.onload = initMap;
</script>
</body>
</html>
'''
# HTMLファイルに書き込む
output_file_path = r"C:\mapion_sc\locations_map.html"
with open(output_file_path, 'w', encoding='utf-8') as f:
f.write(html_content)
print("HTMLファイルが作成されました:", output_file_path)
こんな感じで書いて(Python↑)
↓HTMLはこんな感じ
<!DOCTYPE html>
<html>
<head>
<title>てすてす</title>
<script src="https://maps.googleapis.com/maps/api/js?key=YouareAPIkey"></script>
<style>
#map {
height: 500px;
width: 100%;
}
#info {
margin-top: 10px;
padding: 10px;
border: 1px solid #ccc;
background-color: #f9f9f9;
}
</style>
</head>
<body>
<h1>てすとだ</h1>
<div id="map"></div>
<div id="info">ここに情報が表示されます。</div>
<script>
function initMap() {
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 10,
center: {lat: 35.681236, lng: 139.767125} // 初期位置を東京に設定
});
var locations = [
{
url: "リンク",
title: "タイトル",
lat: 0,
lng: 0
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13207/ILSP0060882948_ipclm/",
title: "松原町コミュニティセンター",
lat: 35.7121036,
lng: 139.3570727
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13207/21331874349/",
title: "昭島市立 松原町高齢者福祉センター",
lat: 35.7146658,
lng: 139.3544556
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13207/ILSP0060892556_ipclm/",
title: "昭島市公民館",
lat: 35.7059102,
lng: 139.3540161
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13207/21330796333/",
title: "昭島市立 市民会館・KOTORIホール",
lat: 35.7134316,
lng: 139.3661483
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13207/21331925211/",
title: "昭島市立 朝日町高齢者福祉センター",
lat: 35.7061888,
lng: 139.3706042
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13207/21330796984/",
title: "拝島三丁目アパート集会室",
lat: 35.711551,
lng: 139.334322
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13207/ILSP0060909632_ipclm/",
title: "福島町公民館",
lat: 36.457509,
lng: 136.468673
},
{
url: "東京都あきる野市の公民館一覧",
title: "",
lat: 0,
lng: 0
},
{
url: "リンク",
title: "タイトル",
lat: 0,
lng: 0
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13228/21331125959/",
title: "あきる野市 中央公民館",
lat: 35.73006580000001,
lng: 139.3023533
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13228/ILSP0060896660_ipclm/",
title: "中央公民館",
lat: 0,
lng: 0
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13228/21330872473/",
title: "瀬戸岡会館",
lat: 35.7382295,
lng: 139.2925999
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13228/21330871652/",
title: "牛沼会館",
lat: 35.7192993,
lng: 139.2854087
},
{
url: "https://www.mapion.co.jp/phonebook/M13007/13228/ILSP0060910533_ipclm/",
title: "城山荘公民館",
lat: 35.72906529999999,
lng: 139.2000813
}
];
locations.forEach(function(location) {
var marker = new google.maps.Marker({
position: {lat: location.lat, lng: location.lng},
map: map,
title: location.title
});
marker.addListener('click', function() {
map.setZoom(15);
map.setCenter(marker.getPosition());
document.getElementById('info').innerHTML =
`<strong>${location.title}</strong><br>
<a href="${location.url}" target="_blank">ホームページ</a>`;
});
});
}
</script>
<script>
window.onload = initMap;
</script>
</body>
</html>
こんな感じで出てきましたええこや
Share this content: